Building Multi-language Support in React Native with GraphQL
Introduction
Creating applications that can seamlessly transition between multiple languages is essential for reaching a global audience. When you combine React Native’s cross-platform capabilities with GraphQL’s flexible data fetching, you can build a powerful internationalization (i18n) system that delivers a personalized experience to users worldwide.
In this article, we’ll explore how to implement multi-language support in a React Native application using GraphQL. We’ll cover server-driven translation strategies, dynamic content localization, RTL layout support, and performance considerations that will help you build a truly global app.
Understanding the Challenges of Internationalization
Before diving into implementation details, it’s important to understand the key challenges of building multi-language applications:
- Static vs. Dynamic Content: Some text is hardcoded in your app, while other content comes from your backend
- Text Expansion: Translations can vary significantly in length (German text is often 30% longer than English)
- Layout Direction: Supporting right-to-left (RTL) languages like Arabic and Hebrew
- Cultural Considerations: Dates, numbers, currencies, and other formatting differences
- Performance Impact: Ensuring translations don’t slow down your application
A well-designed i18n solution with GraphQL addresses these challenges by leveraging the strengths of both technologies.
Setting Up the Foundation: Client-Side i18n
Let’s start with the foundation of our multi-language system. We’ll need a client-side i18n library to handle static text translations within our React Native application.
Installing the Required Packages
yarn add i18next react-i18next @react-native-community/async-storage
Setting Up i18next
Create a configuration file for i18next:
// src/i18n/index.ts
import i18n from 'i18next';
import { initReactI18next } from 'react-i18next';
import AsyncStorage from '@react-native-community/async-storage';
import * as RNLocalize from 'react-native-localize';
// Import your static translation files
import en from './translations/en.json';
import fr from './translations/fr.json';
import ar from './translations/ar.json';
const LANGUAGES = {
en,
fr,
ar
};
const LANGUAGE_DETECTOR = {
type: 'languageDetector',
async: true,
detect: async (callback) => {
// Get stored language from AsyncStorage
const storedLanguage = await AsyncStorage.getItem('APP_LANGUAGE');
if (storedLanguage) {
return callback(storedLanguage);
}
// If no language is stored, use device locale
const deviceLocale = RNLocalize.getLocales()[0];
const languageCode = deviceLocale.languageCode;
// Check if we support this language
const supportedLanguage = Object.keys(LANGUAGES).includes(languageCode) ? languageCode : 'en'; // Default to English
callback(supportedLanguage);
},
init: () => {},
cacheUserLanguage: (language) => {
AsyncStorage.setItem('APP_LANGUAGE', language);
}
};
i18n
.use(LANGUAGE_DETECTOR)
.use(initReactI18next)
.init({
resources: LANGUAGES,
fallbackLng: 'en',
react: {
useSuspense: false
},
interpolation: {
escapeValue: false
}
});
export default i18n;
Creating Translation Files
Create JSON files for each supported language:
// src/i18n/translations/en.json
{
"translation": {
"welcome": "Welcome to our app",
"settings": {
"language": "Language",
"theme": "Theme",
"notifications": "Notifications"
},
"common": {
"loading": "Loading...",
"error": "An error occurred",
"retry": "Try Again"
}
}
}
Using Translations in Components
Now we can use the translations in our React Native components:
import React from 'react';
import { View, Text, Button } from 'react-native';
import { useTranslation } from 'react-i18next';
const WelcomeScreen = () => {
const { t, i18n } = useTranslation();
const changeLanguage = (lng) => {
i18n.changeLanguage(lng);
};
return (
<View style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}>
<Text>{t('welcome')}</Text>
<View style={{ marginTop: 20 }}>
<Button title="English" onPress={() => changeLanguage('en')} />
<Button title="Français" onPress={() => changeLanguage('fr')} />
<Button title="العربية" onPress={() => changeLanguage('ar')} />
</View>
</View>
);
};
export default WelcomeScreen;
Server-Driven Translation Strategies with GraphQL
While the client-side i18n setup handles static text, we need a strategy for dynamic content coming from our GraphQL API. There are several approaches to consider:
1. Language as a Query Parameter
The simplest approach is to include the user’s language preference as a parameter in your GraphQL queries:
query GetArticles($language: String!) {
articles(language: $language) {
id
title
summary
content
}
}
On the server side, you would use this parameter to return content in the requested language:
// Server-side resolver
const resolvers = {
Query: {
articles: async (_, { language }) => {
return db.articles.findAll({
where: { language }
});
}
}
};
2. Field-Level Translations
For more flexibility, you can structure your schema to include translations at the field level:
type Article {
id: ID!
title(language: String): String!
summary(language: String): String!
content(language: String): String!
publishedAt: DateTime!
}
query GetArticles {
articles {
id
title(language: "fr")
summary(language: "fr")
content(language: "fr")
publishedAt
}
}
The resolver would handle each field’s translation:
const resolvers = {
Article: {
title: async (article, { language }) => {
if (!language) return article.title; // Default language
const translation = await db.translations.findOne({
where: {
articleId: article.id,
field: 'title',
language
}
});
return translation ? translation.value : article.title;
}
// Similar resolvers for summary and content
}
};
3. Returning Multiple Translations
Another approach is to return all available translations and let the client decide which to display:
type Translation {
language: String!
value: String!
}
type Article {
id: ID!
titleTranslations: [Translation!]!
summaryTranslations: [Translation!]!
contentTranslations: [Translation!]!
publishedAt: DateTime!
}
query GetArticles {
articles {
id
titleTranslations {
language
value
}
summaryTranslations {
language
value
}
publishedAt
}
}
On the client side, you would select the appropriate translation based on the user’s language preference:
import { useTranslation } from 'react-i18next';
import { useGetArticlesQuery } from '../generated/graphql';
const ArticleList = () => {
const { i18n } = useTranslation();
const { data, loading } = useGetArticlesQuery();
if (loading) return <LoadingIndicator />;
return (
<FlatList
data={data.articles}
renderItem={({ item }) => {
// Find the translation for the current language
const titleTranslation =
item.titleTranslations.find((t) => t.language === i18n.language) ||
item.titleTranslations.find((t) => t.language === 'en'); // Fallback
return <ArticleCard title={titleTranslation.value} publishedAt={item.publishedAt} />;
}}
/>
);
};
Implementing Dynamic Content Localization
Now that we have our basic i18n infrastructure in place, let’s implement a more sophisticated system that handles dynamic content localization.
Creating a Language-Aware Apollo Client
First, we’ll create a custom Apollo Client that automatically includes the user’s language preference in every request:
// src/apollo/client.ts
import { ApolloClient, InMemoryCache, createHttpLink, ApolloLink } from '@apollo/client';
import { setContext } from '@apollo/client/link/context';
import i18n from '../i18n';
// Create an HTTP link
const httpLink = createHttpLink({
uri: 'https://your-graphql-endpoint.com/graphql'
});
// Add language to each request
const languageLink = setContext((_, { headers }) => {
return {
headers: {
...headers,
'Accept-Language': i18n.language
}
};
});
// Create the Apollo Client instance
const client = new ApolloClient({
link: languageLink.concat(httpLink),
cache: new InMemoryCache()
});
export default client;
Setting Up Language-Aware GraphQL Types
Next, let’s create a GraphQL schema that supports localization:
# schema.graphql
enum Language {
EN
FR
AR
ES
DE
}
type LocalizedString {
translations: [Translation!]!
}
type Translation {
language: Language!
text: String!
}
type Product {
id: ID!
name: LocalizedString!
description: LocalizedString!
price: Float!
currency: String!
}
type Query {
products(language: Language): [Product!]!
product(id: ID!, language: Language): Product
}
Implementing the Language Context Provider
Create a React context to manage language state across your app:
// src/contexts/LanguageContext.tsx
import React, { createContext, useState, useEffect, useContext } from 'react';
import { useTranslation } from 'react-i18next';
import AsyncStorage from '@react-native-community/async-storage';
import { I18nManager } from 'react-native';
import * as RNLocalize from 'react-native-localize';
export type Language = {
code: string;
name: string;
isRTL: boolean;
};
export const LANGUAGES: Language[] = [
{ code: 'en', name: 'English', isRTL: false },
{ code: 'fr', name: 'Français', isRTL: false },
{ code: 'ar', name: 'العربية', isRTL: true },
{ code: 'es', name: 'Español', isRTL: false },
{ code: 'de', name: 'Deutsch', isRTL: false }
];
type LanguageContextType = {
currentLanguage: Language;
setLanguage: (language: Language) => Promise<void>;
isRTL: boolean;
};
const LanguageContext = createContext<LanguageContextType | undefined>(undefined);
export const LanguageProvider: React.FC = ({ children }) => {
const { i18n } = useTranslation();
const [currentLanguage, setCurrentLanguage] = useState<Language>(
LANGUAGES.find((lang) => lang.code === i18n.language) || LANGUAGES[0]
);
useEffect(() => {
// Initialize language based on device locale or stored preference
const initLanguage = async () => {
try {
const storedLangCode = await AsyncStorage.getItem('APP_LANGUAGE');
if (storedLangCode) {
const language = LANGUAGES.find((lang) => lang.code === storedLangCode);
if (language) {
await setLanguage(language);
return;
}
}
// Use device locale as fallback
const deviceLocale = RNLocalize.getLocales()[0];
const langCode = deviceLocale.languageCode;
const language = LANGUAGES.find((lang) => lang.code === langCode) || LANGUAGES[0];
await setLanguage(language);
} catch (error) {
console.error('Failed to initialize language:', error);
// Default to English
await setLanguage(LANGUAGES[0]);
}
};
initLanguage();
}, []);
const setLanguage = async (language: Language) => {
try {
// Change i18next language
await i18n.changeLanguage(language.code);
// Handle RTL layout changes
if (I18nManager.isRTL !== language.isRTL) {
I18nManager.forceRTL(language.isRTL);
// Note: In a real app, you might want to restart the app here
// to ensure RTL changes take effect everywhere
}
// Store the selection
await AsyncStorage.setItem('APP_LANGUAGE', language.code);
setCurrentLanguage(language);
} catch (error) {
console.error('Failed to set language:', error);
}
};
return (
<LanguageContext.Provider
value={{
currentLanguage,
setLanguage,
isRTL: currentLanguage.isRTL
}}
>
{children}
</LanguageContext.Provider>
);
};
export const useLanguage = () => {
const context = useContext(LanguageContext);
if (context === undefined) {
throw new Error('useLanguage must be used within a LanguageProvider');
}
return context;
};
Using the Language Context in GraphQL Queries
Now we can use our language context in our GraphQL operations:
import React from 'react';
import { View, Text, FlatList } from 'react-native';
import { useLanguage } from '../contexts/LanguageContext';
import { useTranslation } from 'react-i18next';
import { useProductsQuery } from '../generated/graphql';
const ProductList = () => {
const { currentLanguage } = useLanguage();
const { t } = useTranslation();
const { data, loading, error } = useProductsQuery({
variables: {
language: currentLanguage.code.toUpperCase()
}
});
if (loading) return <Text>{t('common.loading')}</Text>;
if (error) return <Text>{t('common.error')}</Text>;
return (
<FlatList
data={data?.products}
keyExtractor={(item) => item.id}
renderItem={({ item }) => {
// Find the translation for the current language or fall back to English
const nameTranslation =
item.name.translations.find((t) => t.language === currentLanguage.code.toUpperCase()) ||
item.name.translations.find((t) => t.language === 'EN');
const descTranslation =
item.description.translations.find(
(t) => t.language === currentLanguage.code.toUpperCase()
) || item.description.translations.find((t) => t.language === 'EN');
return (
<View style={{ marginBottom: 20 }}>
<Text style={{ fontWeight: 'bold' }}>{nameTranslation?.text}</Text>
<Text>{descTranslation?.text}</Text>
<Text>
{item.price} {item.currency}
</Text>
</View>
);
}}
/>
);
};
export default ProductList;
RTL Layout Support with GraphQL-Aware Components
Supporting right-to-left (RTL) languages like Arabic and Hebrew requires attention to layout direction. Let’s create some GraphQL-aware components that handle RTL automatically:
Creating Direction-Aware Components
// src/components/DirectionalView.tsx
import React from 'react';
import { View, StyleSheet, ViewProps, FlexStyle } from 'react-native';
import { useLanguage } from '../contexts/LanguageContext';
interface DirectionalViewProps extends ViewProps {
row?: boolean;
}
export const DirectionalView: React.FC<DirectionalViewProps> = ({
style,
row = false,
children,
...props
}) => {
const { isRTL } = useLanguage();
const baseStyle: FlexStyle = row
? {
flexDirection: isRTL ? 'row-reverse' : 'row'
}
: {};
return (
<View style={[baseStyle, style]} {...props}>
{children}
</View>
);
};
Creating a Directional Text Component
// src/components/LocalizedText.tsx
import React from 'react';
import { Text, TextProps, StyleSheet, I18nManager } from 'react-native';
import { useLanguage } from '../contexts/LanguageContext';
interface LocalizedTextProps extends TextProps {
text: string;
}
export const LocalizedText: React.FC<LocalizedTextProps> = ({ text, style, ...props }) => {
const { isRTL } = useLanguage();
return (
<Text
style={[
{
textAlign: isRTL ? 'right' : 'left',
writingDirection: isRTL ? 'rtl' : 'ltr'
},
style
]}
{...props}
>
{text}
</Text>
);
};
Using these Components with GraphQL Data
import React from 'react';
import { LocalizedText } from '../components/LocalizedText';
import { DirectionalView } from '../components/DirectionalView';
import { useProductQuery } from '../generated/graphql';
import { useLanguage } from '../contexts/LanguageContext';
const ProductDetail = ({ productId }) => {
const { currentLanguage } = useLanguage();
const { data, loading } = useProductQuery({
variables: {
id: productId,
language: currentLanguage.code.toUpperCase()
}
});
if (loading || !data?.product) return null;
const product = data.product;
// Find translation for current language
const nameTranslation =
product.name.translations.find((t) => t.language === currentLanguage.code.toUpperCase()) ||
product.name.translations.find((t) => t.language === 'EN');
const descTranslation =
product.description.translations.find(
(t) => t.language === currentLanguage.code.toUpperCase()
) || product.description.translations.find((t) => t.language === 'EN');
return (
<DirectionalView style={{ padding: 16 }}>
<LocalizedText
text={nameTranslation.text}
style={{ fontSize: 20, fontWeight: 'bold', marginBottom: 8 }}
/>
<LocalizedText text={descTranslation.text} style={{ marginBottom: 16 }} />
<DirectionalView row style={{ justifyContent: 'space-between' }}>
<LocalizedText text="Price:" />
<Text>
{product.price} {product.currency}
</Text>
</DirectionalView>
</DirectionalView>
);
};
export default ProductDetail;
Performance Considerations for Multi-language Apps
Implementing multi-language support can potentially impact your app’s performance. Here are some strategies to maintain high performance:
1. Memoize Translated Components
Use React’s useMemo
to prevent unnecessary re-renders when translations don’t change:
import React, { useMemo } from 'react';
import { useTranslation } from 'react-i18next';
const TranslatedContent = ({ productId }) => {
const { t, i18n } = useTranslation();
// Only re-run this expensive operation when language changes
const content = useMemo(() => {
// Complex content generation that depends on translations
return {
title: t('product.title'),
description: t('product.description')
// ... more translations
};
}, [t, i18n.language]);
return (
<View>
<Text>{content.title}</Text>
<Text>{content.description}</Text>
</View>
);
};
2. Optimize GraphQL Queries with Fragments
Use GraphQL fragments to fetch only the languages you need:
fragment ProductFields on Product {
id
name {
translations(languages: [$language, "EN"]) {
language
text
}
}
description {
translations(languages: [$language, "EN"]) {
language
text
}
}
price
currency
}
query GetProduct($id: ID!, $language: Language!) {
product(id: $id) {
...ProductFields
}
}
3. Cache Translations Locally
Use Apollo Client’s cache to store translations:
const cache = new InMemoryCache({
typePolicies: {
LocalizedString: {
keyFields: false,
fields: {
translations: {
// Merge incoming translations with existing ones
merge(existing = [], incoming) {
// Create a map of existing translations by language
const translationMap = new Map();
existing.forEach((item) => {
translationMap.set(item.language, item);
});
// Update with incoming translations
incoming.forEach((item) => {
translationMap.set(item.language, item);
});
// Convert back to array
return Array.from(translationMap.values());
}
}
}
}
}
});
4. Implement Language Bundles
Split your translations into bundles and load them dynamically:
const loadLanguageBundle = async (language) => {
let translations;
switch (language) {
case 'fr':
translations = await import('./translations/fr.json');
break;
case 'ar':
translations = await import('./translations/ar.json');
break;
case 'es':
translations = await import('./translations/es.json');
break;
default:
translations = await import('./translations/en.json');
}
i18n.addResourceBundle(language, 'translation', translations.default);
};
5. Lazy Load Secondary Languages
Only load the primary language at startup, and load others on demand:
import i18n from 'i18next';
import { initReactI18next } from 'react-i18next';
import AsyncStorage from '@react-native-community/async-storage';
import * as RNLocalize from 'react-native-localize';
// Only import English at startup
import en from './translations/en.json';
i18n.use(initReactI18next).init({
resources: {
en: {
translation: en
}
},
lng: 'en',
fallbackLng: 'en',
interpolation: {
escapeValue: false
}
});
// Get stored or device language
const getInitialLanguage = async () => {
const storedLanguage = await AsyncStorage.getItem('APP_LANGUAGE');
if (storedLanguage && storedLanguage !== 'en') {
// Dynamically load the stored language
await loadLanguageBundle(storedLanguage);
i18n.changeLanguage(storedLanguage);
} else if (!storedLanguage) {
// Check device language
const deviceLanguage = RNLocalize.getLocales()[0].languageCode;
if (deviceLanguage !== 'en') {
await loadLanguageBundle(deviceLanguage);
i18n.changeLanguage(deviceLanguage);
}
}
};
// Initialize
getInitialLanguage();
export default i18n;
Conclusion
Implementing multi-language support in a React Native application with GraphQL offers tremendous flexibility and power. By leveraging both client-side i18n libraries and GraphQL’s schema capabilities, you can create a seamless, performant international user experience.
The approaches outlined in this article provide a comprehensive foundation for supporting multiple languages in your React Native app:
- Client-side static text translations with i18n
- Server-driven dynamic content localization via GraphQL
- RTL layout support with direction-aware components
- Performance optimizations to ensure smooth user experience
By following these patterns, you can deliver a truly global application that resonates with users worldwide, regardless of their language preference or reading direction. As mobile apps continue to expand globally, robust internationalization becomes not just a nice feature, but an essential requirement for success.
Remember that internationalization is not just about translation—it’s about creating an experience that feels natural and native to users in every supported locale. With the power of React Native and GraphQL, you have all the tools you need to make that happen.
Subscribe now!
Get latest news in regards to tech.
We wont spam you. Promise.
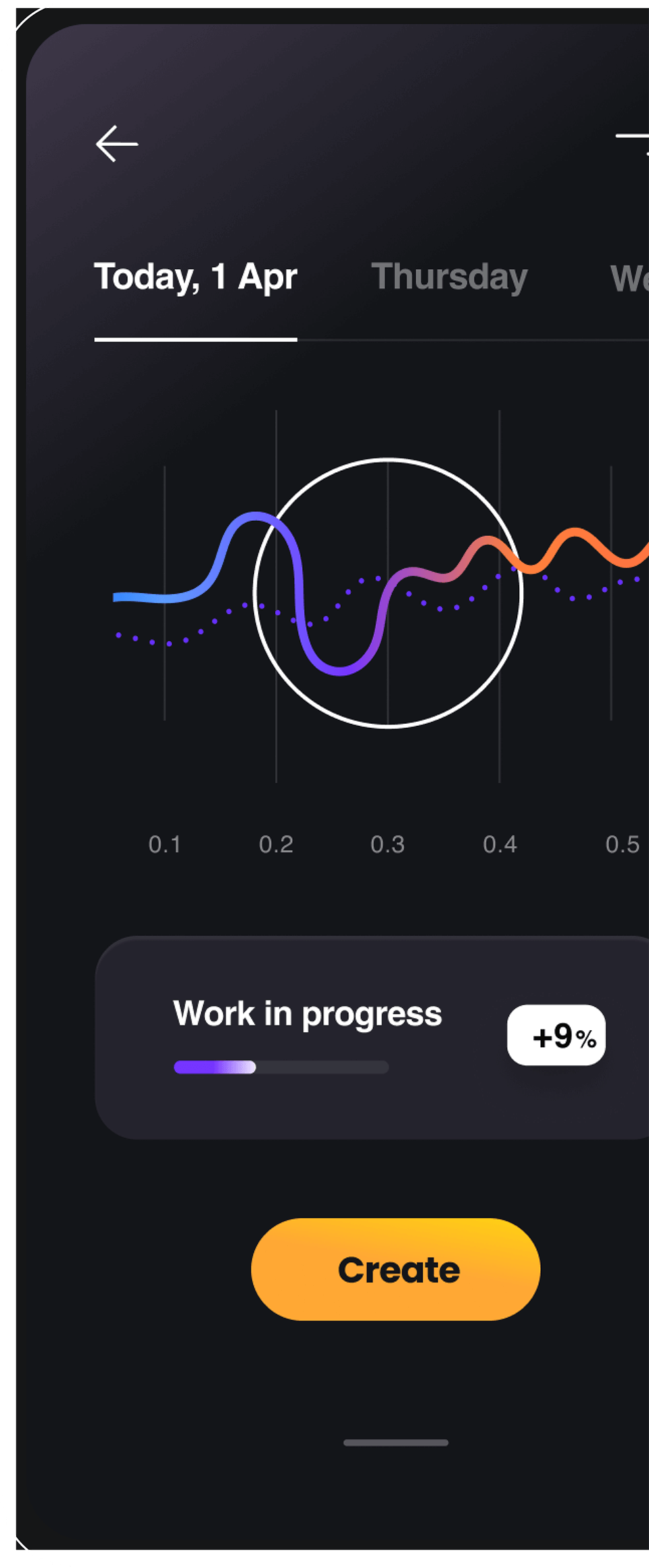
© 2025 Otherside Limited. All rights reserved.