Integrating HyperPay with React Native in 2024: A Comprehensive Guide
Integrating HyperPay with React Native in 2024: A Comprehensive Guide
Introduction
Payment gateways are a crucial component of any e-commerce or service-based application. In the Middle East and North Africa region, HyperPay has emerged as one of the leading payment service providers, offering secure and efficient payment processing solutions. In this guide, I’ll walk you through the process of integrating HyperPay with a React Native application, based on my experience implementing it in a real-world project.
What is HyperPay?
HyperPay is a payment gateway that supports various payment methods, including Visa, Mastercard, MADA, Apple Pay, and more. It provides a secure environment for processing payments and is compliant with PCI DSS standards.
Prerequisites
Before we begin, make sure you have:
- A HyperPay merchant account
- Access to the HyperPay dashboard
- A React Native project set up
- Basic understanding of React Native and native modules
- HyperPay SDKs for Android and iOS
Obtaining the HyperPay SDKs
To integrate HyperPay into your React Native application, you’ll need to obtain the official SDKs:
For Android:
- Contact HyperPay support to get access to the Android SDK
- You’ll receive a
.aar
file that needs to be included in your project - Place the
.aar
file in your project’sandroid/app/libs/
directory
For iOS:
- Contact HyperPay support to get access to the iOS SDK
- You’ll receive the SDK package with necessary frameworks
- The SDK can be integrated via CocoaPods or manual installation
Project Structure
Our implementation will consist of three main parts:
- Native Modules: We’ll create native modules for Android and iOS to communicate with the HyperPay SDK.
- React Native Hook: We’ll create a custom hook to interact with the native modules.
- Integration Layer: We’ll create an integration layer to handle API calls and payment flows.
Step 1: Setting Up Native Modules
Android Implementation
First, let’s set up the Android native module for HyperPay.
- Add the HyperPay SDK to your project:
- Add the
.aar
file to theandroid/app/libs/
directory - Update your app’s
build.gradle
file to include the HyperPay SDK:
- Add the
dependencies {
// Other dependencies
implementation fileTree(dir: 'libs', include: ['*.aar'])
// Or specify the exact file
// implementation files('libs/oppwa.mobile-4.x.x.aar')
}
- Create the HyperPay module and package files:
// android/app/src/main/java/com/yourpackage/hyperpay/HyperPayModule.kt
package com.yourpackage.hyperpay
import android.app.Activity
import android.content.Context
import android.content.Intent
import android.content.SharedPreferences
import com.facebook.react.bridge.*
import com.yourpackage.hyperpay.activity.CheckoutUIActivity
class HyperPayModule(reactContext: ReactApplicationContext) : ReactContextBaseJavaModule(reactContext) {
private var checkoutPromise: Promise? = null
private val PREFS_NAME = "HyperPayPrefs"
private val TOKEN_KEY = "token"
private var successCallback: Callback? = null
private var errorCallback: Callback? = null
// Activity event listener to handle payment results
private val activityEventListener: ActivityEventListener = object : BaseActivityEventListener() {
override fun onActivityResult(activity: Activity, requestCode: Int, resultCode: Int, data: Intent?) {
if (requestCode == CheckoutUIActivity.REQUEST_CODE) {
if (resultCode == Activity.RESULT_OK && data != null) {
val paymentResult = data.getStringExtra(CheckoutUIActivity.PAYMENT_RESULT)
val paymentMethod = data.getStringExtra(CheckoutUIActivity.PAYMENT_METHOD)
val resultData = Arguments.createMap()
resultData.putString("checkoutID", paymentResult)
resultData.putString("paymentBrand", paymentMethod)
resultData.putString("resourcePath", "")
successCallback?.invoke(resultData)
} else {
errorCallback?.invoke("Payment was cancelled by the user")
}
successCallback = null
errorCallback = null
}
}
}
init {
reactContext.addActivityEventListener(activityEventListener)
}
override fun getName(): String {
return "HyperPayModule"
}
@ReactMethod
fun openCheckoutUI(params: ReadableMap, successCallback: Callback, errorCallback: Callback) {
val currentActivity = currentActivity
if (currentActivity == null) {
errorCallback.invoke("Activity Error: Current activity is null")
return
}
try {
val checkoutId = params.getString("checkoutId")
val token = params.getString("token")
val isSandbox = params.getString("is_sandbox")
// Store values in SharedPreferences if needed
val sharedPreferences: SharedPreferences = currentActivity.getSharedPreferences(PREFS_NAME, Context.MODE_PRIVATE)
val editor: SharedPreferences.Editor = sharedPreferences.edit()
editor.putString(TOKEN_KEY, token)
editor.apply()
// Launch checkout activity
val intent = Intent(currentActivity, CheckoutUIActivity::class.java)
intent.putExtra(CheckoutUIActivity.EXTRA_CHECKOUT_ID, checkoutId)
intent.putExtra(CheckoutUIActivity.EXTRA_LANGUAGE, "en")
currentActivity.startActivityForResult(intent, CheckoutUIActivity.REQUEST_CODE)
this.successCallback = successCallback
this.errorCallback = errorCallback
} catch (e: Exception) {
errorCallback.invoke(e.message)
}
}
}
- Create the HyperPay package:
// android/app/src/main/java/com/yourpackage/hyperpay/HyperPayPackage.kt
package com.yourpackage.hyperpay
import com.facebook.react.ReactPackage
import com.facebook.react.bridge.NativeModule
import com.facebook.react.bridge.ReactApplicationContext
import com.facebook.react.uimanager.ViewManager
class HyperPayPackage : ReactPackage {
override fun createNativeModules(reactContext: ReactApplicationContext): List<NativeModule> {
return listOf(HyperPayModule(reactContext))
}
override fun createViewManagers(reactContext: ReactApplicationContext): List<ViewManager<*, *>> {
return emptyList()
}
}
- Create the CheckoutUIActivity to handle the payment process:
// android/app/src/main/java/com/yourpackage/hyperpay/activity/CheckoutUIActivity.kt
package com.yourpackage.hyperpay.activity
import android.app.Activity
import android.content.Intent
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
import com.oppwa.mobile.connect.checkout.dialog.CheckoutActivity
import com.oppwa.mobile.connect.checkout.meta.CheckoutSettings
import com.oppwa.mobile.connect.exception.PaymentError
import com.oppwa.mobile.connect.provider.Connect
import com.oppwa.mobile.connect.provider.Transaction
import com.oppwa.mobile.connect.provider.TransactionType
class CheckoutUIActivity : AppCompatActivity() {
companion object {
const val REQUEST_CODE = 1
const val PAYMENT_RESULT = "payment_result"
const val PAYMENT_METHOD = "payment_method"
const val EXTRA_CHECKOUT_ID = "checkout_id"
const val EXTRA_LANGUAGE = "language"
}
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
val checkoutId = intent.getStringExtra(EXTRA_CHECKOUT_ID) ?: ""
val language = intent.getStringExtra(EXTRA_LANGUAGE) ?: "en"
// Configure checkout settings
val checkoutSettings = CheckoutSettings(
checkoutId,
Connect.ProviderMode.TEST, // Change to LIVE for production
language
)
// Set payment brands
checkoutSettings.paymentBrands = listOf("VISA", "MASTER", "MADA")
// Set shopper result URL (for 3D Secure)
checkoutSettings.shopperResultUrl = "yourapp://result"
// Start the checkout UI
val intent = Intent(this, CheckoutActivity::class.java)
intent.putExtra(CheckoutActivity.EXTRA_CHECKOUT_SETTINGS, checkoutSettings)
startActivityForResult(intent, CheckoutActivity.REQUEST_CODE)
}
override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) {
super.onActivityResult(requestCode, resultCode, data)
if (requestCode == CheckoutActivity.REQUEST_CODE) {
when (resultCode) {
CheckoutActivity.RESULT_OK -> {
// Transaction completed
val transaction: Transaction = data?.getParcelableExtra(CheckoutActivity.CHECKOUT_RESULT_TRANSACTION)!!
val paymentMethod = transaction.paymentParams.paymentBrand
// Check transaction type
if (transaction.transactionType == TransactionType.SYNC) {
// Handle synchronous transaction
val resultIntent = Intent()
resultIntent.putExtra(PAYMENT_RESULT, transaction.resourcePath)
resultIntent.putExtra(PAYMENT_METHOD, paymentMethod)
setResult(Activity.RESULT_OK, resultIntent)
finish()
} else {
// Asynchronous transaction - handle accordingly
// This might involve waiting for the payment to be confirmed
}
}
CheckoutActivity.RESULT_CANCELED -> {
// User canceled the payment
setResult(Activity.RESULT_CANCELED)
finish()
}
CheckoutActivity.RESULT_ERROR -> {
// Error occurred during checkout
val error: PaymentError = data?.getParcelableExtra(CheckoutActivity.CHECKOUT_RESULT_ERROR)!!
setResult(Activity.RESULT_CANCELED)
finish()
}
}
}
}
}
- Register the module in MainApplication.kt:
// android/app/src/main/java/com/yourpackage/MainApplication.kt
import com.yourpackage.hyperpay.HyperPayPackage
// Inside the getPackages() method
override fun getPackages(): List<ReactPackage> {
return listOf(
MainReactPackage(),
// Other packages...
HyperPayPackage()
)
}
- Update your AndroidManifest.xml to include the CheckoutUIActivity:
<!-- android/app/src/main/AndroidManifest.xml -->
<activity
android:name=".hyperpay.activity.CheckoutUIActivity"
android:configChanges="orientation|keyboardHidden|screenSize"
android:windowSoftInputMode="adjustResize" />
iOS Implementation
For iOS, we need to create a native module to interact with the OPPWAMobile SDK:
First, add the HyperPay SDK to your iOS project:
- The SDK can be added manually by downloading it from HyperPay
- Alternatively, you can add it to your Podfile, but you’ll need access to the private repository
Create the header file:
// ios/YourApp/HyperPayModule.h
#import <React/RCTBridgeModule.h>
#import <React/RCTEventEmitter.h>
#import <OPPWAMobile/OPPWAMobile.h>
@interface HyperPayModule : RCTEventEmitter <RCTBridgeModule, OPPCheckoutProviderDelegate>
@end
- Then, implement the module:
// ios/YourApp/HyperPayModule.m
#import "HyperPayModule.h"
#import <React/RCTLog.h>
#import <React/RCTConvert.h>
#import <OPPWAMobile/OPPWAMobile.h>
@interface HyperPayModule () <OPPCheckoutProviderDelegate>
@end
@implementation HyperPayModule {
RCTResponseSenderBlock onDoneClick;
RCTResponseSenderBlock onCancelClick;
OPPPaymentProvider *provider;
OPPCheckoutProvider *checkoutProvider;
NSDictionary *indic;
OPPTransaction *currentTransaction;
}
RCT_EXPORT_MODULE();
RCT_EXPORT_METHOD(openCheckoutUI:(NSDictionary *)inputIndic
doneCallback:(RCTResponseSenderBlock)doneCallback
cancelCallback:(RCTResponseSenderBlock)cancelCallback) {
onDoneClick = doneCallback;
onCancelClick = cancelCallback;
indic = inputIndic;
// Set provider mode based on sandbox flag
if ([indic[@"is_sandbox"] isEqualToString:@"1"]) {
provider = [OPPPaymentProvider paymentProviderWithMode:OPPProviderModeTest];
} else {
provider = [OPPPaymentProvider paymentProviderWithMode:OPPProviderModeLive];
}
// Configure checkout settings
OPPCheckoutSettings *checkoutSettings = [[OPPCheckoutSettings alloc] init];
checkoutSettings.paymentBrands = @[@"VISA", @"MASTER", @"MADA"];
checkoutSettings.shopperResultURL = @"yourapp://result";
// Create checkout provider
checkoutProvider = [OPPCheckoutProvider checkoutProviderWithPaymentProvider:provider checkoutID:indic[@"checkoutId"] settings:checkoutSettings];
checkoutProvider.delegate = self;
// Present checkout UI
dispatch_async(dispatch_get_main_queue(), ^{
[checkoutProvider presentCheckoutForSubmittingTransactionCompletionHandler:^(OPPTransaction * _Nullable transaction, NSError * _Nullable error) {
if (error) {
onCancelClick(@[@"cancel"]);
} else if (transaction.type == OPPTransactionTypeSynchronous) {
NSDictionary *responseDic = @{
@"checkoutID": transaction.paymentParams.checkoutID,
@"paymentBrand": transaction.paymentParams.paymentBrand,
@"resourcePath": transaction.resourcePath
};
onDoneClick(@[responseDic]);
} else if (transaction.type == OPPTransactionTypeAsynchronous) {
currentTransaction = transaction;
[[NSNotificationCenter defaultCenter] addObserver:self
selector:@selector(didReceiveAsynchronousPaymentCallback)
name:@"AsyncPaymentCompletedNotification"
object:nil];
}
} cancelHandler:^{
onCancelClick(@[@"cancel"]);
}];
});
}
// Delegate methods
- (void)checkoutProvider:(OPPCheckoutProvider *)checkoutProvider didFinishTransaction:(OPPTransaction *)transaction completedWithError:(NSError *)error {
if (error) {
onCancelClick(@[error.localizedDescription]);
} else {
NSString *checkoutID = transaction.paymentParams.checkoutID;
NSString *paymentBrand = transaction.paymentParams.paymentBrand;
NSDictionary *response = @{
@"checkoutID": checkoutID,
@"paymentBrand": paymentBrand,
@"resourcePath": transaction.resourcePath
};
onDoneClick(@[response]);
}
}
// Handle asynchronous payment callbacks
- (void)didReceiveAsynchronousPaymentCallback {
[[NSNotificationCenter defaultCenter] removeObserver:self
name:@"AsyncPaymentCompletedNotification"
object:nil];
[checkoutProvider dismissCheckoutAnimated:YES completion:^{
dispatch_async(dispatch_get_main_queue(), ^{
if (currentTransaction) {
NSString *checkoutID = currentTransaction.paymentParams.checkoutID;
NSString *paymentBrand = currentTransaction.paymentParams.paymentBrand;
NSDictionary *response = @{
@"checkoutID": checkoutID,
@"paymentBrand": paymentBrand,
@"resourcePath": currentTransaction.resourcePath
};
onDoneClick(@[response]);
currentTransaction = nil;
}
});
}];
}
@end
- Register the module in your app delegate:
// ios/YourApp/AppDelegate.m
#import "AppDelegate.h"
#import "HyperPayModule.h"
// Add the module to your app
- Update your Podfile to link the required frameworks:
# ios/Podfile
# Note: The HyperPay SDK should be provided to you by HyperPay support
# You'll need to integrate it according to their instructions
# Example of manual linking:
target 'YourApp' do
# Other pods...
# If you have received direct access to the SDK repository:
# pod 'OPPWAMobile', :git => '[private-repository-url]', :tag => '[version]'
# If you're manually installing the SDK:
# Copy the framework files to an 'SDKs' folder in your project and link them
end
Step 2: Creating a React Native Hook for HyperPay
Now, let’s create a custom hook to interface with our native modules:
// src/hooks/useHyperPay.ts
import { NativeModules } from 'react-native';
interface HyperPayConfig {
checkoutId: string;
isSandbox?: boolean;
additionalParams?: Record<string, unknown>;
}
interface HyperPayResponse {
checkoutID: string;
paymentBrand: string;
resourcePath: string;
}
interface HyperPayHook {
initiatePayment: (config: HyperPayConfig) => Promise<HyperPayResponse>;
}
export const useHyperPay = (): HyperPayHook => {
const { HyperPayModule } = NativeModules;
const initiatePayment = (config: HyperPayConfig) => {
return new Promise<HyperPayResponse>((resolve, reject) => {
if (!HyperPayModule) {
reject(new Error('HyperPay module is not initialized'));
return;
}
HyperPayModule.openCheckoutUI(
{
checkoutId: config.checkoutId,
token: config.additionalParams?.token || '',
is_sandbox: config.isSandbox ? '1' : '0',
...config.additionalParams
},
(response: HyperPayResponse) => {
resolve(response);
},
(error: unknown) => {
reject(error);
}
);
});
};
return {
initiatePayment
};
};
Step 3: Creating a Payment Trigger Function
Next, let’s create a function to trigger payments from our React Native application:
// src/services/paymentService.ts
import { useHyperPay } from '../hooks/useHyperPay';
export const createPaymentService = () => {
const { initiatePayment } = useHyperPay();
const initializePayment = async (amount: number) => {
try {
// Make a request to your backend to initialize payment
const response = await fetch('https://your-api.com/api/initialize-payment', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
Authorization: 'Bearer YOUR_AUTH_TOKEN'
},
body: JSON.stringify({ amount })
});
if (!response.ok) {
throw new Error('Failed to initialize payment');
}
const data = await response.json();
return data.data; // { checkoutId, referenceId }
} catch (error) {
console.error('Payment initialization failed:', error);
throw error;
}
};
const processPayment = async (amount: number) => {
try {
// Step 1: Initialize payment with backend
const paymentData = await initializePayment(amount);
// Step 2: Launch HyperPay payment flow
const result = await initiatePayment({
checkoutId: paymentData.checkoutId,
isSandbox: true // Change to false for production
});
// Step 3: Verify payment status (optional)
const verificationResult = await verifyPayment(result.checkoutID);
return {
success: true,
referenceId: paymentData.referenceId,
verificationResult
};
} catch (error) {
console.error('Payment processing failed:', error);
return {
success: false,
error: error.message
};
}
};
const verifyPayment = async (checkoutId: string) => {
try {
const response = await fetch(`https://your-api.com/api/payment-status/${checkoutId}`, {
headers: {
Authorization: 'Bearer YOUR_AUTH_TOKEN'
}
});
if (!response.ok) {
throw new Error('Failed to verify payment');
}
return response.json();
} catch (error) {
console.error('Payment verification failed:', error);
throw error;
}
};
return {
processPayment,
verifyPayment
};
};
Step 4: Using the Payment Service in Your Component
Now, let’s use the payment service in a React Native component:
// src/screens/Checkout.tsx
import React, { useState } from 'react';
import { View, Button, Text, StyleSheet, Alert } from 'react-native';
import { createPaymentService } from '../services/paymentService';
import { useNavigation } from '@react-navigation/native';
const CheckoutScreen = () => {
const navigation = useNavigation();
const [isLoading, setIsLoading] = useState(false);
const paymentService = createPaymentService();
const handlePayment = async () => {
setIsLoading(true);
try {
// Amount in the smallest currency unit (e.g., cents for USD)
const amount = 1000; // $10.00
const result = await paymentService.processPayment(amount);
if (result.success) {
// Payment was successful
console.log('Payment successful', result);
// Navigate to success screen
navigation.navigate('PaymentSuccess', {
referenceId: result.referenceId,
amount
});
} else {
// Payment failed
Alert.alert('Payment Failed', result.error || 'Unknown error occurred');
}
} catch (error) {
console.error('Payment error:', error);
Alert.alert('Payment Error', 'An unexpected error occurred');
} finally {
setIsLoading(false);
}
};
return (
<View style={styles.container}>
<Text style={styles.title}>Checkout</Text>
<Text style={styles.amount}>Amount: $10.00</Text>
<Button
title={isLoading ? 'Processing...' : 'Pay Now'}
onPress={handlePayment}
disabled={isLoading}
/>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
padding: 20,
justifyContent: 'center',
alignItems: 'center'
},
title: {
fontSize: 24,
fontWeight: 'bold',
marginBottom: 20
},
amount: {
fontSize: 18,
marginBottom: 30
}
});
export default CheckoutScreen;
Step 5: Backend Integration
For a complete implementation, you’ll need a backend that can interact with HyperPay’s server-side APIs. Here’s a simplified example of what your backend API might look like:
// Backend API (example in Node.js with Express)
const express = require('express');
const axios = require('axios');
const router = express.Router();
// Initialize payment
router.post('/initialize-payment', async (req, res) => {
try {
const { amount } = req.body;
// Your HyperPay credentials
const merchantId = process.env.HYPERPAY_MERCHANT_ID;
const accessToken = process.env.HYPERPAY_ACCESS_TOKEN;
const entityId = process.env.HYPERPAY_ENTITY_ID;
const baseUrl = process.env.HYPERPAY_BASE_URL;
// Create a payment request
const response = await axios.post(
`${baseUrl}/v1/checkouts`,
new URLSearchParams({
entityId,
amount,
currency: 'SAR',
paymentType: 'DB'
}).toString(),
{
headers: {
Authorization: `Bearer ${accessToken}`,
'Content-Type': 'application/x-www-form-urlencoded'
}
}
);
// Return checkout ID to the client
res.json({
success: true,
data: {
checkoutId: response.data.id,
referenceId: response.data.ndc || Date.now().toString() // or generate your own reference ID
}
});
} catch (error) {
console.error('Payment initialization failed:', error.response?.data || error.message);
res.status(500).json({
success: false,
message: 'Failed to initialize payment'
});
}
});
// Verify payment status
router.get('/payment-status/:checkoutId', async (req, res) => {
try {
const { checkoutId } = req.params;
// Your HyperPay credentials
const merchantId = process.env.HYPERPAY_MERCHANT_ID;
const accessToken = process.env.HYPERPAY_ACCESS_TOKEN;
const entityId = process.env.HYPERPAY_ENTITY_ID;
const baseUrl = process.env.HYPERPAY_BASE_URL;
// Check payment status
const response = await axios.get(
`${baseUrl}/v1/checkouts/${checkoutId}/payment?entityId=${entityId}`,
{
headers: {
Authorization: `Bearer ${accessToken}`
}
}
);
res.json({
success: true,
data: response.data
});
} catch (error) {
console.error('Payment status check failed:', error.response?.data || error.message);
res.status(500).json({
success: false,
message: 'Failed to check payment status'
});
}
});
module.exports = router;
Common Challenges and Troubleshooting
1. Handling 3D Secure Authentication
For 3D Secure payments, you need to properly handle the callback URL:
For Android:
<!-- android/app/src/main/AndroidManifest.xml -->
<activity
android:name="com.oppwa.mobile.connect.checkout.dialog.RedirectActivity"
android:theme="@style/Theme.Oppwa.Transparent">
<intent-filter>
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.DEFAULT" />
<category android:name="android.intent.category.BROWSABLE" />
<data android:scheme="yourapp" />
</intent-filter>
</activity>
For iOS, add to your Info.plist:
<key>CFBundleURLTypes</key>
<array>
<dict>
<key>CFBundleURLName</key>
<string>yourapp</string>
<key>CFBundleURLSchemes</key>
<array>
<string>yourapp</string>
</array>
</dict>
</array>
2. Handling Different Payment Methods
Different payment brands may require specific configurations. For example, MADA cards have specific BIN ranges and validation rules:
// Helper function to identify MADA cards
const isMADA = (cardNumber: string): boolean => {
const madaBins = [
'440647',
'440795',
'446404',
'457865',
'457997',
'474491',
'474492',
'474493',
'588845',
'588846',
'588847',
'588848',
'588849',
'588850',
'588851',
'588852',
'588853',
'588854',
'588855',
'493428',
'504300',
'508141',
'508142',
'508143',
'508146',
'508149',
'508251',
'528047',
'528049',
'535825',
'543357',
'557606',
'557607',
'557608',
'557609',
'557610',
'557612',
'589005',
'589206',
'604906',
'605141',
'636120',
'968201',
'968202',
'968203',
'968204',
'968205',
'968206',
'968207',
'968208',
'968209',
'968210',
'968211'
];
return madaBins.some((bin) => cardNumber.startsWith(bin));
};
3. Error Handling
Implement proper error handling in your application:
try {
await initiatePayment({
checkoutId,
isSandbox: true
});
} catch (error) {
// Handle different types of errors
if (error.message.includes('canceled')) {
// User canceled the payment
console.log('Payment was canceled by the user');
} else if (error.message.includes('network')) {
// Network error
console.log('Network error, please try again');
} else {
// General error
console.log(`Payment failed: ${error.message}`);
}
}
Best Practices
Sandbox Testing: Always test thoroughly in the sandbox environment before going live.
Security: Never store sensitive payment information on your mobile application.
Error Handling: Implement comprehensive error handling to provide a smooth user experience.
Logging: Implement logging for debugging but ensure you don’t log sensitive information.
UI/UX: Design a user-friendly payment flow that aligns with your application’s design language.
Validation: Implement client-side validation for payment forms to improve user experience.
Testing: Test your implementation across different devices, operating systems, and network conditions.
Conclusion
Conclusion
Integrating HyperPay with React Native requires significant effort in setting up native modules for both Android and iOS platforms, but the result is a seamless payment experience that can significantly improve your app’s user experience. By following this comprehensive guide, you should be able to implement a complete payment solution that works across both major mobile platforms.
Key Integration Steps
- Creating native modules that communicate with the HyperPay SDK
- Building a JavaScript bridge through React Native hooks
- Implementing proper error handling and payment verification
Maintenance and Testing
While the initial setup might seem complex, the modular approach outlined in this guide allows for easier maintenance and future updates. Remember to:
- Thoroughly test your implementation in the sandbox environment before moving to production
- Always follow security best practices when handling payment information
Benefits of HyperPay
By leveraging HyperPay’s comprehensive payment infrastructure, you can offer your users:
- A wide range of payment options
- Industry-standard security
- Reliable payment processing
Additional Resources
For more detailed information and the latest updates, refer to the official HyperPay documentation.
Happy coding, and may your payment integrations be smooth and secure!
Subscribe now!
Get latest news in regards to tech.
We wont spam you. Promise.
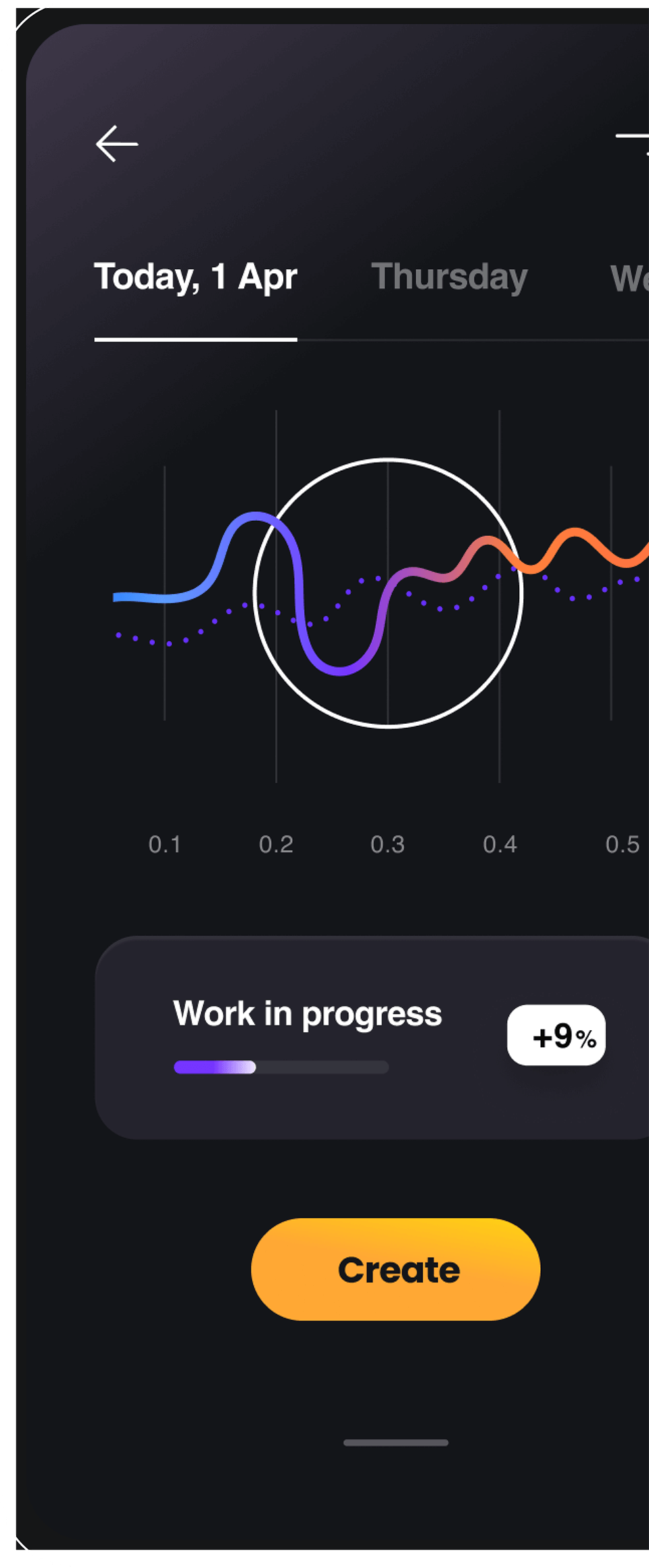
© 2025 Otherside Limited. All rights reserved.