Monorepo Architecture with Turborepo: A Practical Guide
Introduction
In modern web development, managing multiple related packages and applications can become complex very quickly. Monorepos offer a solution to this complexity by housing all your code in a single repository. This approach provides several benefits, including shared code, simplified dependencies, and coordinated builds.
Among the many tools available for managing monorepos, Turborepo has emerged as a powerful option, especially for JavaScript and TypeScript projects. In this guide, we’ll explore how to set up and optimize a monorepo using Turborepo.
What is a Monorepo?
A monorepo (monolithic repository) is a version control strategy where multiple projects or applications are stored in a single repository. This differs from the traditional approach of having separate repositories for each project.
Benefits of monorepos include:
- Shared code: Easy sharing of code between applications
- Simplified dependency management: Single version of dependencies across projects
- Atomic commits: Changes across multiple packages in a single commit
- Coordinated building and testing: Build and test related packages together
Why Choose Turborepo?
Turborepo is a high-performance build system for JavaScript and TypeScript codebases. It was designed specifically for monorepos and offers several key advantages:
- Intelligent caching: Automatically caches task outputs for faster builds
- Parallel execution: Runs tasks in parallel for maximum efficiency
- Incremental builds: Only rebuilds what changed
- Remote caching: Share build caches with your team or CI/CD pipeline
- Zero configuration: Works out of the box with minimal setup
Setting Up a Monorepo with Turborepo
Initial Setup
Let’s start by creating a new monorepo using Turborepo:
npx create-turbo@latest
This command sets up a basic monorepo structure with the following components:
- A root
package.json
file that defines workspaces - A
turbo.json
configuration file - Example apps and packages
Understanding the Structure
A typical Turborepo monorepo structure looks like this:
my-monorepo/
├── apps/ # Consumer applications
│ ├── web/ # Next.js app
│ └── api/ # Express API
├── packages/ # Shared packages
│ ├── ui/ # UI component library
│ ├── config/ # Shared configuration
│ └── database/ # Database client and models
├── package.json # Root package.json
└── turbo.json # Turborepo configuration
Configuring Workspaces
In your root package.json
, define your workspaces:
{
"name": "my-monorepo",
"private": true,
"workspaces": ["apps/*", "packages/*"],
"devDependencies": {
"turbo": "^2.0.0"
}
}
Configuring Turborepo
Create a turbo.json
file in the root of your repository:
{
"$schema": "https://turbo.build/schema.json",
"pipeline": {
"build": {
"dependsOn"0: ["^build"],
"outputs": ["dist/**", ".next/**"]
},
"lint": {
"outputs": []
},
"dev": {
"cache": false,
"persistent": true
}
}
}
This configuration defines:
- A
build
task that depends on the build tasks of all dependencies - A
lint
task that produces no outputs - A
dev
task that isn’t cached and runs persistently
Managing Dependencies Between Packages
Creating Shared Packages
One of the key benefits of a monorepo is the ability to share code between applications. Let’s create a shared UI package:
mkdir -p packages/ui
cd packages/ui
npm init -y
Edit the packages/ui/package.json
:
{
"name": "@my-project/ui",
"version": "0.0.1",
"main": "./dist/index.js",
"types": "./dist/index.d.ts",
"scripts": {
"build": "tsup src/index.tsx --format cjs,esm --dts",
"dev": "tsup src/index.tsx --format cjs,esm --watch --dts",
"lint": "eslint "src/**/*.ts*""
},
"devDependencies": {
"tsup": "^6.5.0",
"typescript": "^5.0.0"
},
"peerDependencies": {
"react": "^18.0.0"
}
}
Using Shared Packages in Apps
Now you can use this shared package in your applications:
cd apps/web
npm install @my-project/ui
In your app, import components from the shared package:
// apps/web/src/app/page.tsx
import { Button } from '@my-project/ui';
export default function Page() {
return (
<div>
<h1>Welcome to My App</h1>
<Button>Click me</Button>
</div>
);
}
Optimizing Build and Development Workflows
Parallel Execution
Turborepo automatically executes tasks in parallel when possible. You can run tasks across all workspaces:
npx turbo run build
Caching for Faster Builds
Turborepo’s intelligent caching system stores the outputs of tasks and reuses them when inputs haven’t changed. This dramatically speeds up builds:
# First build (slow)
npx turbo run build
# Second build (fast, uses cache)
npx turbo run build
Remote Caching
For team collaboration, set up remote caching:
npx turbo login
npx turbo link
This allows team members to share build caches, dramatically improving build times across the team.
Advanced Configurations
Custom Pipeline Configurations
You can define custom configurations for specific packages:
{
"$schema": "https://turbo.build/schema.json",
"pipeline": {
"build": {
"dependsOn": ["^build"],
"outputs": ["dist/**", ".next/**"]
},
"apps/web#build": {
"dependsOn": ["^build"],
"outputs": [".next/**"],
"env": ["NEXT_PUBLIC_API_URL"]
}
}
}
Environment Variables
Configure environment variables for specific tasks:
{
"pipeline": {
"build": {
"env": ["NODE_ENV", "API_KEY"]
}
}
}
Best Practices
Consistent Versioning
Maintain consistent versioning across packages using tools like Changesets:
npm install -D @changesets/cli
npx changeset init
Standardized Scripts
Keep script names consistent across packages to simplify workflow commands:
build
: Build the packagedev
: Start development modelint
: Run lintingtest
: Run testsclean
: Clean build artifacts
Organized Dependencies
- Use
dependencies
for runtime dependencies - Use
devDependencies
for build-time dependencies - Use
peerDependencies
for framework dependencies
Conclusion
Setting up a monorepo with Turborepo provides a powerful foundation for managing complex JavaScript and TypeScript projects. By centralizing your code, sharing components and configurations, and optimizing build processes, you can significantly improve your development workflow and team collaboration.
The key benefits include faster builds through intelligent caching, simplified dependency management, and easier code sharing between applications. As your project grows, these advantages become increasingly valuable, making Turborepo an excellent choice for modern JavaScript applications.
In future posts, we’ll explore more advanced topics like integrating CI/CD pipelines, managing deployments, and setting up testing frameworks in a Turborepo monorepo.
Subscribe now!
Get latest news in regards to tech.
We wont spam you. Promise.
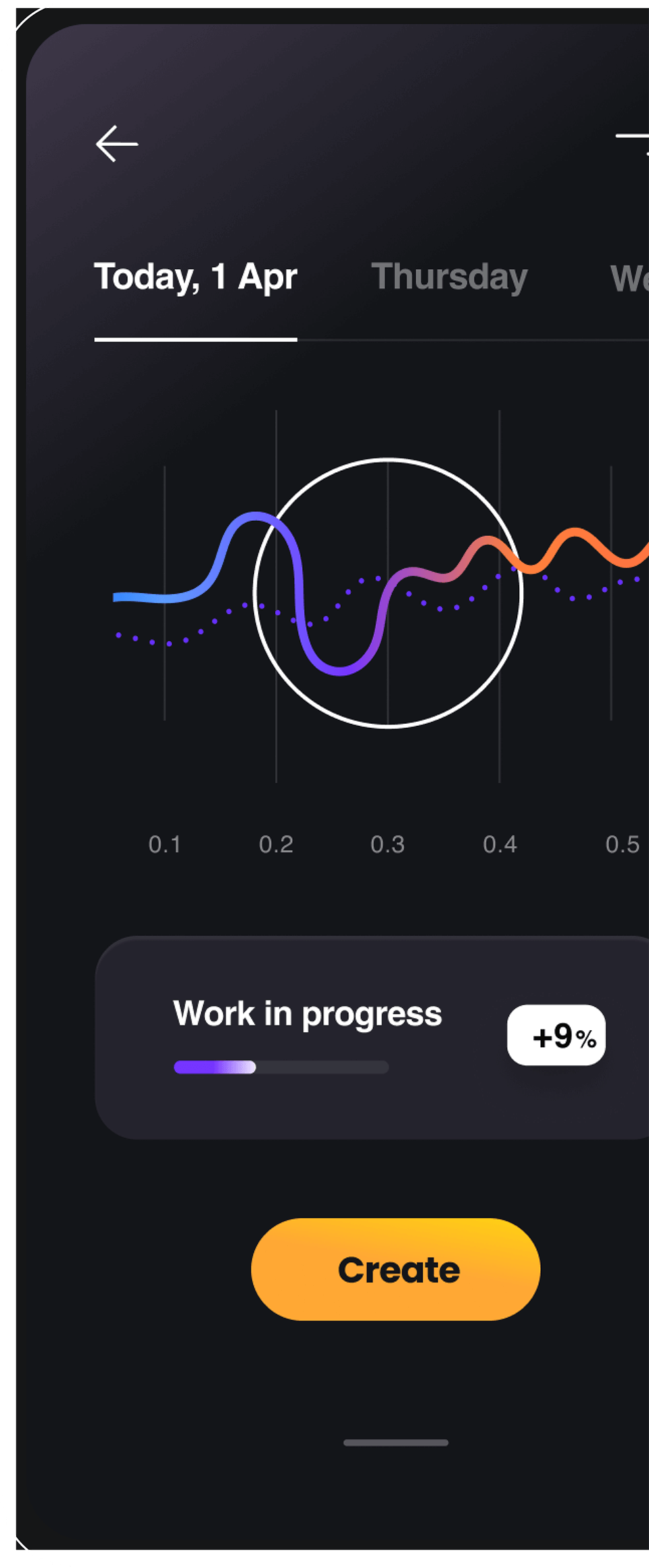
© 2025 Otherside Limited. All rights reserved.