Setting Up GraphQL Code Generator in React Native: A Complete Guide
1. Introduction
GraphQL has revolutionized how developers interact with APIs, offering a more efficient and flexible alternative to traditional REST APIs. For mobile developers working with React Native, GraphQL provides powerful benefits: precise data fetching, reduced over-fetching, and a strongly-typed schema that clearly defines available data.
While GraphQL itself brings many advantages, managing the interface between your GraphQL schema and your TypeScript code can become tedious and error-prone when done manually. This is where GraphQL Code Generator (graphql-codegen) comes into play.
In this comprehensive guide, we’ll walk through how to set up and leverage GraphQL Code Generator in a React Native application. We’ll cover everything from initial installation to advanced usage patterns, helping you build type-safe, efficient, and maintainable mobile applications.
By the end of this article, you’ll understand how to:
- Configure GraphQL Code Generator in a React Native project
- Generate TypeScript types from your GraphQL schema
- Create typed React hooks for your queries and mutations
- Integrate code generation into your development workflow
- Implement best practices for organizing your GraphQL operations
Let’s dive in and see how GraphQL Code Generator can transform your React Native development experience.
2. Understanding GraphQL Code Generator
What is GraphQL Code Generator?
GraphQL Code Generator is a tool that generates code from your GraphQL schema and operations. While its primary function for React Native developers is generating TypeScript types and React hooks, it’s actually a versatile plugin-based platform that can generate many kinds of output—from TypeScript interfaces to Rust code to documentation.
At its core, GraphQL Code Generator takes two inputs:
- Your GraphQL schema (defining the structure of available data)
- Your GraphQL operations (queries, mutations, and subscriptions)
It then processes these inputs through plugins to produce code that’s ready to use in your application.
Benefits of Using GraphQL Code Generator in React Native
Type Safety
Perhaps the most significant benefit is full end-to-end type safety. When you use the generated types and hooks:
- Your IDE will show errors if you try to access fields that don’t exist
- TypeScript will catch type mismatches during compilation
- You’ll get autocomplete suggestions for available fields
- Type refinements happen automatically based on conditional fields
This dramatically reduces runtime errors and improves code quality.
Developer Experience
The generated code provides a seamless developer experience:
- No need to manually write TypeScript interfaces for your API responses
- IDE autocomplete suggests available fields as you write code
- React hooks handle query execution, loading states, and caching
- Changes to your schema or operations automatically propagate to your code
Consistency
GraphQL Code Generator ensures consistency between your client code and server schema:
- If the server schema changes, your generated code will reflect those changes
- If you try to use fields that don’t exist, you’ll get immediate feedback
- The same operation always produces consistent types throughout your application
Reduced Boilerplate
Without code generation, you’d need to write significant boilerplate:
- TypeScript interfaces for every query response
- TypeScript interfaces for all input variables
- Hooks or functions to execute the queries
- Logic to handle loading and error states
GraphQL Code Generator handles all this for you, letting you focus on your application’s business logic instead.
3. Getting Started: Project Setup
Required Dependencies
To get started with GraphQL Code Generator in your React Native project, you’ll need to install several packages:
yarn add @apollo/client graphql
yarn add -D @graphql-codegen/cli @graphql-codegen/typescript @graphql-codegen/typescript-operations @graphql-codegen/typescript-react-apollo
Let’s break down what each package does:
@apollo/client
: The Apollo Client library for handling GraphQL requestsgraphql
: The core GraphQL JavaScript implementation@graphql-codegen/cli
: The command-line interface for GraphQL Code Generator@graphql-codegen/typescript
: Plugin for generating TypeScript types from your schema@graphql-codegen/typescript-operations
: Plugin for generating TypeScript types for your operations@graphql-codegen/typescript-react-apollo
: Plugin for generating React hooks for your operations
Basic Project Structure
For optimal organization, consider structuring your GraphQL files like this:
src/
├── graphql/
│ ├── authentication.gql # Auth-related queries/mutations
│ ├── user.gql # User-related queries/mutations
│ ├── listing.gql # Listing-related queries/mutations
│ └── ... # Other domain-specific files
├── generated/
│ └── graphql.ts # Generated TypeScript code
└── ... # Your React Native components
This structure keeps your GraphQL operations organized by domain, making them easier to manage as your application grows.
Setting up Apollo Client
Before we configure GraphQL Code Generator, let’s set up Apollo Client in your React Native application:
// src/apollo/client.ts
import { ApolloClient, InMemoryCache, createHttpLink } from '@apollo/client';
import { setContext } from '@apollo/client/link/context';
import AsyncStorage from '@react-native-async-storage/async-storage';
// Create an HTTP link
const httpLink = createHttpLink({
uri: 'https://your-graphql-endpoint.com/graphql'
});
// Auth link to attach tokens to requests
const authLink = setContext(async (_, { headers }) => {
// Get the authentication token from AsyncStorage
const token = await AsyncStorage.getItem('authToken');
// Return the headers to the context
return {
headers: {
...headers,
authorization: token ? `Bearer ${token}` : ''
}
};
});
// Create the Apollo Client instance
const client = new ApolloClient({
link: authLink.concat(httpLink),
cache: new InMemoryCache()
});
export default client;
Now, wrap your React Native application with the Apollo Provider:
// App.tsx
import React from 'react';
import { ApolloProvider } from '@apollo/client';
import client from './src/apollo/client';
import AppNavigator from './src/navigation';
const App = () => {
return (
<ApolloProvider client={client}>
<AppNavigator />
</ApolloProvider>
);
};
export default App;
With Apollo Client set up, we’re ready to configure GraphQL Code Generator.
4. Configuring GraphQL Code Generator
Creating the codegen.yml Configuration File
GraphQL Code Generator is configured through a YAML file, typically named codegen.yml
. Create this file in the root of your project:
overwrite: true
schema: 'https://your-graphql-endpoint.com/graphql'
documents: 'src/graphql/**/*.gql'
generates:
src/generated/graphql.ts:
plugins:
- 'typescript'
- 'typescript-operations'
- 'typescript-react-apollo'
config:
withHooks: true
withComponent: false
withHOC: false
skipTypename: false
reactApolloVersion: 3
Let’s break down this configuration:
overwrite
: Ensures the generated file is overwritten on each runschema
: The URL of your GraphQL schema (can also be a local file path)documents
: Glob pattern for your GraphQL operation filesgenerates
: Specifies the output files and their configurationssrc/generated/graphql.ts
: The path where the generated code will be savedplugins
: The list of plugins to use for code generationconfig
: Configuration options for the plugins
Understanding Available Plugins
The three main plugins we’re using are:
- typescript: Generates basic TypeScript types for your GraphQL schema
- typescript-operations: Generates TypeScript types for your specific operations
- typescript-react-apollo: Generates React hooks for your operations
Each plugin serves a specific purpose in the code generation pipeline:
- The
typescript
plugin creates interfaces for all types in your schema - The
typescript-operations
plugin creates specific types for your queries and mutations - The
typescript-react-apollo
plugin creates React hooks that use these types
Plugin Configuration Options
The config
section allows you to customize how the plugins generate code:
withHooks: true
: Generate React hooks (e.g.,useMyQuery
)withComponent: false
: Don’t generate React componentswithHOC: false
: Don’t generate higher-order componentsskipTypename: false
: Include__typename
field in generated typesreactApolloVersion: 3
: Target Apollo Client version 3.x
You can adjust these options based on your project’s needs. For React Native applications, the configuration above is generally optimal.
5. Organizing GraphQL Operations
Best Practices for Structure
When organizing your GraphQL operations, consider these best practices:
- Group by domain: Keep related operations together (e.g., all user-related queries in one file)
- Name your operations: Always name your queries and mutations for better debugging
- Use fragments for shared fields: Define reusable fragments for common fields
- Keep operations focused: Each operation should fetch only what it needs
Here’s an example of well-organized GraphQL operations:
# src/graphql/user.gql
fragment UserFields on User {
id
name
email
profileImage
}
query GetUserProfile {
currentUser {
...UserFields
phoneNumber
accountCreatedAt
}
}
mutation UpdateUserProfile($input: UpdateUserInput!) {
updateUser(input: $input) {
...UserFields
updatedAt
}
}
Using Fragments for Reusability
Fragments are reusable pieces of GraphQL queries. They help you:
- Avoid repeating field selections
- Ensure consistency across queries
- Make your queries more readable
- Enable co-location of field requirements with components
For example, if multiple queries need user details, define a fragment once and reuse it:
fragment UserFields on User {
id
username
fullName
profilePicture {
url
width
height
}
}
query GetUserPosts {
userPosts {
id
title
content
author {
...UserFields
}
}
}
query GetUserComments {
userComments {
id
text
post {
id
title
}
author {
...UserFields
}
}
}
This approach ensures consistency and reduces redundancy in your code.
Organizing by Module/Feature
For larger applications, consider organizing your GraphQL files by feature or module:
src/
├── features/
│ ├── authentication/
│ │ ├── graphql/
│ │ │ ├── login.gql
│ │ │ ├── register.gql
│ │ │ └── fragments.gql
│ │ └── components/
│ ├── profile/
│ │ ├── graphql/
│ │ │ ├── profile.gql
│ │ │ ├── edit-profile.gql
│ │ │ └── fragments.gql
│ │ └── components/
│ └── ...
This structure keeps related code together, making it easier to navigate and maintain your codebase as it grows.
6. Running the Code Generator
Setting Up npm/yarn Scripts
Add GraphQL Code Generator commands to your package.json
scripts:
"scripts": {
"generate": "graphql-codegen --config codegen.yml",
"generate:watch": "graphql-codegen --config codegen.yml --watch",
"prestart": "yarn generate",
"start": "react-native start"
}
These scripts allow you to:
- Run code generation manually with
yarn generate
- Run code generation in watch mode with
yarn generate:watch
- Automatically generate code before starting the React Native dev server
Integrating with Your Development Workflow
There are several ways to integrate code generation into your workflow:
- Pre-start generation: As shown above, run generation before starting the dev server
- Watch mode: Keep generation running in a separate terminal with
yarn generate:watch
- Git hooks: Use husky to run generation on git hooks (e.g., pre-commit)
- CI/CD pipeline: Verify generated code is up to date in your CI/CD pipeline
For most developers, a combination of pre-start generation and watch mode provides the best experience.
Automating Generation on Project Start
The prestart
script ensures code is always generated before you start development:
"prestart": "yarn generate",
"start": "react-native start"
This approach prevents issues where developers forget to regenerate code after pulling changes.
For a more comprehensive approach, you could also add:
"prebuild": "yarn generate",
"build": "react-native build-android && react-native build-ios"
This ensures your production builds always use the latest generated code.
7. Using Generated Types and Hooks
Working with Generated TypeScript Types
The generated code provides TypeScript types for all your GraphQL operations:
// Generated types example
export type GetUserProfileQuery = {
__typename?: 'Query';
currentUser?: {
__typename?: 'User';
id: string;
name: string;
email: string;
profileImage?: string | null;
phoneNumber?: string | null;
accountCreatedAt: any;
} | null;
};
export type UpdateUserProfileMutation = {
__typename?: 'Mutation';
updateUser: {
__typename?: 'User';
id: string;
name: string;
email: string;
profileImage?: string | null;
updatedAt: any;
};
};
You can use these types directly in your components:
import { GetUserProfileQuery } from '../generated/graphql';
// Using the generated type
const renderUserInfo = (data: GetUserProfileQuery) => {
if (!data.currentUser) return null;
return (
<View>
<Text>{data.currentUser.name}</Text>
<Text>{data.currentUser.email}</Text>
</View>
);
};
Using the Generated React Hooks
For each query and mutation, GraphQL Code Generator creates a React hook:
// For a query named GetUserProfile
export const useGetUserProfileQuery = (
options?: Omit<UseQueryOptions<GetUserProfileQuery, Error>, 'queryKey'> & { queryKey?: QueryKey }
) => {
return useQuery<GetUserProfileQuery, Error>(
options?.queryKey ?? ['GetUserProfile'],
fetcher<GetUserProfileQuery>(),
options
);
};
// For a mutation named UpdateUserProfile
export const useUpdateUserProfileMutation = () => {
return useMutation<UpdateUserProfileMutation, Error, UpdateUserProfileMutationVariables>(
'UpdateUserProfile',
(variables?: UpdateUserProfileMutationVariables) =>
fetcher<UpdateUserProfileMutation, UpdateUserProfileMutationVariables>(variables)()
);
};
Using these hooks in your components is straightforward:
import React from 'react';
import { View, Text, Button } from 'react-native';
import { useGetUserProfileQuery, useUpdateUserProfileMutation } from '../generated/graphql';
const UserProfileScreen = () => {
// Query hook
const { data, loading, error } = useGetUserProfileQuery();
// Mutation hook
const [updateProfile, { loading: updating }] = useUpdateUserProfileMutation();
if (loading) return <Text>Loading...</Text>;
if (error) return <Text>Error: {error.message}</Text>;
if (!data?.currentUser) return <Text>No user found</Text>;
const handleUpdateName = () => {
updateProfile({
variables: {
input: {
name: 'New Name'
}
}
});
};
return (
<View>
<Text>Name: {data.currentUser.name}</Text>
<Text>Email: {data.currentUser.email}</Text>
<Button title="Update Name" onPress={handleUpdateName} disabled={updating} />
</View>
);
};
export default UserProfileScreen;
Handling Loading, Error, and Data States
The generated hooks provide loading, error, and data states:
const { data, loading, error } = useGetUserProfileQuery();
if (loading) {
return <LoadingIndicator />;
}
if (error) {
return <ErrorDisplay message={error.message} />;
}
if (!data || !data.currentUser) {
return <EmptyState message="No user data available" />;
}
// Safe to use data.currentUser here
return <UserProfile user={data.currentUser} />;
For mutations, you get similar state handling:
const [updateProfile, { loading, error, data }] = useUpdateUserProfileMutation();
// Show loading state in the button
<Button
title={loading ? 'Updating...' : 'Update Profile'}
disabled={loading}
onPress={() => updateProfile({ variables: { input } })}
/>;
// Show error if it exists
{
error && <ErrorMessage message={error.message} />;
}
// Show success message if data exists
{
data && <SuccessMessage message="Profile updated successfully!" />;
}
This state handling makes it easy to create responsive and user-friendly interfaces.
8. Advanced Usage Patterns
Customizing the Output
GraphQL Code Generator offers extensive customization options. Here are some useful configurations:
generates:
src/generated/graphql.ts:
plugins:
- 'typescript'
- 'typescript-operations'
- 'typescript-react-apollo'
config:
# Customize naming conventions
enumsAsTypes: true
constEnums: true
# Customize imports
importOperationTypesFrom: 'Operations'
importDocumentNodeExternallyFrom: 'near-operation-file'
# Add custom scalars mappings
scalars:
Date: 'string'
DateTime: 'Date'
JSON: 'Record<string, any>'
# Customize hooks behavior
pureMagicComment: true
withRefetchFn: true
These options help you tailor the generated code to your project’s specific needs.
Working with Fragments
For complex applications, fragment management becomes crucial. You can organize fragments in separate files and import them:
# fragments/user.gql
fragment UserBasic on User {
id
name
}
fragment UserDetailed on User {
...UserBasic
email
phone
address {
street
city
country
}
}
Then import and use these fragments in your queries:
# queries/userQueries.gql
#import "../fragments/user.gql"
query GetUserList {
users {
...UserBasic
}
}
query GetUserDetail($id: ID!) {
user(id: $id) {
...UserDetailed
}
}
This approach helps keep your GraphQL operations DRY and maintainable.
Handling Complex Queries and Mutations
For complex queries with many parameters, the generated types help ensure correctness:
const { data } = useSearchListingsQuery({
variables: {
input: {
categories: ['ELECTRONICS', 'BOOKS'],
priceRange: { min: 10, max: 1000 },
location: {
lat: 37.7749,
lng: -122.4194,
radius: 10
},
sortBy: 'PRICE_LOW_TO_HIGH',
pagination: {
page: 1,
limit: 20
}
}
}
});
TypeScript will ensure all required fields are provided and with the correct types.
9. Performance Considerations
Managing Bundle Size
GraphQL Code Generator can generate large files, which may impact your bundle size. Consider these strategies:
Split your generated files: Generate multiple smaller files instead of one large file
generates: src/generated/types.ts: plugins: - 'typescript' src/generated/operations/: preset: near-operation-file presetConfig: extension: .generated.tsx baseTypesPath: ../types.ts plugins: - 'typescript-operations' - 'typescript-react-apollo'
Use near-operation-file preset: Generate operation-specific code next to your query files
Set
skipTypename: true
: Remove__typename
fields if you don’t use themUse code splitting: Ensure React Native’s code splitting can separate unused operations
Optimizing GraphQL Operations
To optimize your GraphQL operations:
- Request only what you need: Avoid over-fetching fields
- Use pagination: For large lists, implement cursor-based pagination
- Consider fragments: Use fragments to standardize field selection
- Batch queries: Use Apollo’s query batching for related queries
Caching Strategies
Apollo Client provides powerful caching capabilities:
const client = new ApolloClient({
link: authLink.concat(httpLink),
cache: new InMemoryCache({
typePolicies: {
User: {
fields: {
// Customize how fields are merged/cached
friends: {
merge(existing = [], incoming) {
return [...existing, ...incoming];
}
}
}
}
}
})
});
Consider implementing cache persistence for React Native:
import { AsyncStorageWrapper, persistCache } from 'apollo3-cache-persist';
import AsyncStorage from '@react-native-async-storage/async-storage';
import { InMemoryCache } from '@apollo/client';
const cache = new InMemoryCache();
// Set up cache persistence
persistCache({
cache,
storage: new AsyncStorageWrapper(AsyncStorage)
}).then(() => {
// Continue with Apollo Client setup
const client = new ApolloClient({
link: authLink.concat(httpLink),
cache
});
// Initialize your app
});
This approach improves offline experience and reduces unnecessary network requests.
10. Testing and Debugging
Testing Apollo Components
To test components that use generated hooks:
import { MockedProvider } from '@apollo/client/testing';
import { render, waitFor, screen } from '@testing-library/react-native';
import { GET_USER_PROFILE } from '../graphql/queries';
import UserProfileScreen from './UserProfileScreen';
const mocks = [
{
request: {
query: GET_USER_PROFILE
},
result: {
data: {
currentUser: {
id: '1',
name: 'John Doe',
email: 'john@example.com'
}
}
}
}
];
test('renders user profile when data is loaded', async () => {
render(
<MockedProvider mocks={mocks} addTypename={false}>
<UserProfileScreen />
</MockedProvider>
);
// Initially should show loading
expect(screen.getByText(/loading/i)).toBeTruthy();
// Wait for query to resolve
await waitFor(() => {
expect(screen.getByText(/john doe/i)).toBeTruthy();
expect(screen.getByText(/john@example.com/i)).toBeTruthy();
});
});
Debugging GraphQL Operations
To debug GraphQL operations:
Enable Apollo Client devtools: Use the Apollo Client Devtools extension
Add debug logging: Configure the Apollo link chain to log requests
import { ApolloLink } from '@apollo/client'; const debugLink = new ApolloLink((operation, forward) => { console.log(`[GraphQL Request] ${operation.operationName}`, { variables: operation.variables }); return forward(operation).map((result) => { console.log(`[GraphQL Result] ${operation.operationName}`, { data: result.data, errors: result.errors }); return result; }); }); const client = new ApolloClient({ link: ApolloLink.from([debugLink, authLink, httpLink]), cache: new InMemoryCache() });
Network inspection: Use tools like Flipper or React Native Debugger to inspect network requests
Mock Server Setup
For development without a backend, consider setting up a mock GraphQL server:
import { ApolloClient, InMemoryCache } from '@apollo/client';
import { SchemaLink } from '@apollo/client/link/schema';
import { makeExecutableSchema } from '@graphql-tools/schema';
// Your schema definition
const typeDefs = `
type User {
id: ID!
name: String!
email: String!
}
type Query {
currentUser: User
}
`;
// Your resolvers
const resolvers = {
Query: {
currentUser: () => ({
id: '1',
name: 'Test User',
email: 'test@example.com'
})
}
};
// Create executable schema
const schema = makeExecutableSchema({ typeDefs, resolvers });
// Create Apollo Client with schema link
const client = new ApolloClient({
link: new SchemaLink({ schema }),
cache: new InMemoryCache()
});
This approach allows you to develop and test your UI without a backend dependency.
11. Real-World Example: Implementing a Feature
Let’s implement a complete feature using GraphQL Code Generator: a user profile screen with edit functionality.
Step 1: Define GraphQL Operations
# src/graphql/user.gql
fragment UserFields on User {
id
name
email
bio
profileImage
}
query GetUserProfile {
currentUser {
...UserFields
}
}
mutation UpdateUserProfile($input: UpdateUserInput!) {
updateUser(input: $input) {
...UserFields
}
}
Step 2: Run Code Generation
yarn generate
This will create the necessary types and hooks in src/generated/graphql.ts
.
Step 3: Create the Profile Component
// src/screens/ProfileScreen.tsx
import React, { useState } from 'react';
import { View, Text, TextInput, Button, Image, StyleSheet } from 'react-native';
import { useGetUserProfileQuery, useUpdateUserProfileMutation } from '../generated/graphql';
const ProfileScreen = () => {
const { data, loading, error } = useGetUserProfileQuery();
const [updateProfile, { loading: updating }] = useUpdateUserProfileMutation();
const [isEditing, setIsEditing] = useState(false);
const [name, setName] = useState('');
const [bio, setBio] = useState('');
// Initialize form when data loads
React.useEffect(() => {
if (data?.currentUser) {
setName(data.currentUser.name);
setBio(data.currentUser.bio || '');
}
}, [data]);
if (loading) return <Text style={styles.message}>Loading profile...</Text>;
if (error) return <Text style={styles.error}>Error: {error.message}</Text>;
if (!data?.currentUser) return <Text style={styles.message}>No user data found</Text>;
const handleSave = async () => {
try {
await updateProfile({
variables: {
input: {
name,
bio
}
}
});
setIsEditing(false);
} catch (err) {
console.error('Failed to update profile:', err);
}
};
return (
<View style={styles.container}>
{data.currentUser.profileImage && (
<Image source={{ uri: data.currentUser.profileImage }} style={styles.profileImage} />
)}
{isEditing ? (
<View style={styles.form}>
<TextInput style={styles.input} value={name} onChangeText={setName} placeholder="Name" />
<TextInput
style={[styles.input, styles.bioInput]}
value={bio}
onChangeText={setBio}
placeholder="Bio"
multiline
/>
<View style={styles.buttonRow}>
<Button title="Cancel" onPress={() => setIsEditing(false)} disabled={updating} />
<Button
title={updating ? 'Saving...' : 'Save'}
onPress={handleSave}
disabled={updating}
/>
</View>
</View>
) : (
<View style={styles.profileInfo}>
<Text style={styles.name}>{data.currentUser.name}</Text>
<Text style={styles.email}>{data.currentUser.email}</Text>
{data.currentUser.bio && <Text style={styles.bio}>{data.currentUser.bio}</Text>}
<Button title="Edit Profile" onPress={() => setIsEditing(true)} />
</View>
)}
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
padding: 20,
alignItems: 'center'
},
profileImage: {
width: 100,
height: 100,
borderRadius: 50,
marginBottom: 20
},
profileInfo: {
alignItems: 'center',
width: '100%'
},
name: {
fontSize: 24,
fontWeight: 'bold',
marginBottom: 10
},
email: {
fontSize: 16,
color: '#666',
marginBottom: 20
},
bio: {
fontSize: 16,
textAlign: 'center',
marginBottom: 30
},
form: {
width: '100%'
},
input: {
borderWidth: 1,
borderColor: '#ccc',
borderRadius: 5,
padding: 10,
marginBottom: 15
},
bioInput: {
height: 100,
textAlignVertical: 'top'
},
buttonRow: {
flexDirection: 'row',
justifyContent: 'space-around',
marginTop: 10
},
message: {
fontSize: 18,
marginTop: 20
},
error: {
fontSize: 18,
color: 'red',
marginTop: 20
}
});
export default ProfileScreen;
Step 4: Add Cache Updates
For a better user experience, update the Apollo cache after mutation:
const [updateProfile] = useUpdateUserProfileMutation({
update(cache, { data }) {
if (!data?.updateUser) return;
// Update the cache with the new user data
cache.writeQuery({
query: GetUserProfileDocument,
data: { currentUser: data.updateUser }
});
}
});
This example demonstrates how to use the generated hooks and types to create a fully functional feature with proper loading, error handling, and optimistic UI updates.
12. Conclusion
GraphQL Code Generator transforms how you work with GraphQL in React Native applications by:
- Ensuring type safety across your application
- Reducing boilerplate code with generated hooks and types
- Improving developer experience with IDE autocomplete and compile-time validation
- Maintaining consistency between your client and server
- Supporting complex workflows with advanced configuration options
By integrating GraphQL Code Generator into your development workflow, you’ll spend less time writing repetitive code and more time building features that matter to your users. The tool’s plugin-based architecture allows it to adapt to a wide range of use cases, from small applications to complex enterprise systems.
As your app grows, you’ll appreciate the safeguards that the generated types provide, helping you catch errors early in the development process rather than in production. And when your GraphQL schema evolves, the code generator ensures your client code stays in sync, reducing the risk of runtime errors.
Whether you’re building a new React Native application or integrating GraphQL into an existing one, GraphQL Code Generator provides a solid foundation for type-safe, maintainable, and efficient GraphQL operations. The investment in setting up proper code generation pays dividends throughout your application’s lifecycle, making it an essential tool for modern React Native development.
Start integrating GraphQL Code Generator into your workflow today, and experience the benefits of type-safe GraphQL operations in your React Native applications.
Subscribe now!
Get latest news in regards to tech.
We wont spam you. Promise.
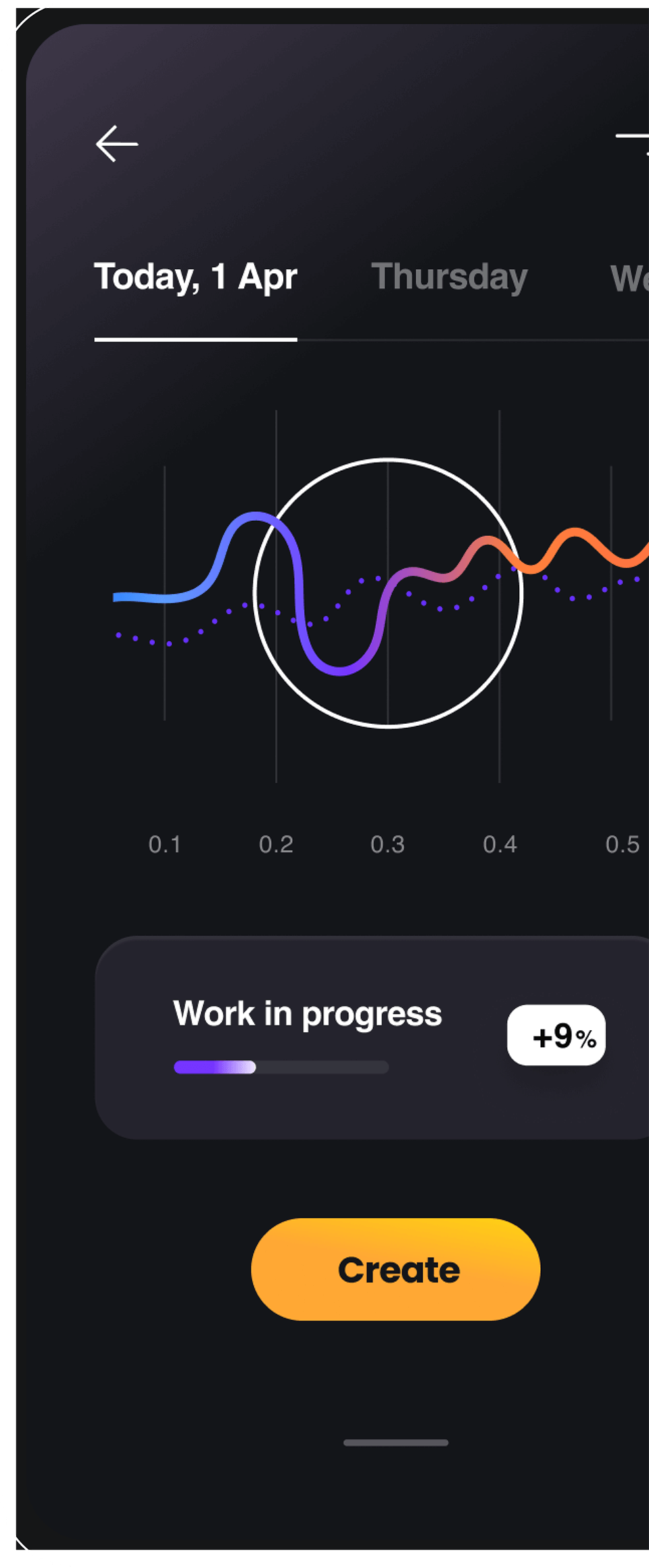
© 2025 Otherside Limited. All rights reserved.