Setting Up NativeWind with Dark Mode in React Native
Introduction
NativeWind brings the power of Tailwind CSS to React Native, allowing you to use utility classes for styling your mobile applications. This guide will walk you through setting up NativeWind, configuring themes including dark mode, and implementing a theme switcher in your React Native application.
Installation
First, let’s install NativeWind and its dependencies:
# Install NativeWind
yarn add nativewind
yarn add --dev tailwindcss
# Initialize Tailwind CSS configuration
npx tailwindcss init
Configuration
Configure Tailwind CSS
Update your tailwind.config.js
file to include the content paths and define your theme:
// tailwind.config.js
module.exports = {
content: ['./App.{js,jsx,ts,tsx}', './src/**/*.{js,jsx,ts,tsx}'],
theme: {
extend: {
colors: {
// Create dynamic theme colors using CSS variables
primary: 'rgb(var(--color-primary) / <alpha-value>)',
secondary: 'rgb(var(--color-secondary) / <alpha-value>)',
background: 'rgb(var(--color-background) / <alpha-value>)',
text: 'rgb(var(--color-text) / <alpha-value>)'
}
}
},
plugins: [
// Set default values for CSS variables
({ addBase }) =>
addBase({
':root': {
'--color-primary': '0 122 255', // iOS blue
'--color-secondary': '236 236 236', // Light gray
'--color-background': '255 255 255', // White
'--color-text': '0 0 0' // Black
}
})
]
};
Configure Babel
Update your babel.config.js
to include the NativeWind plugin:
// babel.config.js
module.exports = {
presets: ['module:@react-native/babel-preset'],
plugins: ['nativewind/babel']
};
Creating Utility Functions
Create a utility file to handle classnames and theme switching:
// src/core/utilities/tailwind.tsx
import { type ClassValue, clsx } from 'clsx';
import { twMerge } from 'tailwind-merge';
import { vars, useColorScheme } from 'nativewind';
// Utility function to combine and merge classnames
export const cn = (...inputs: ClassValue[]): string => {
return twMerge(clsx(inputs));
};
// Define theme variables
export const themes = {
light: vars({
'--color-primary': '0 122 255', // iOS blue
'--color-secondary': '236 236 236', // Light gray
'--color-background': '255 255 255', // White
'--color-text': '0 0 0' // Black
}),
dark: vars({
'--color-primary': '10 132 255', // iOS blue (dark mode)
'--color-secondary': '44 44 46', // Dark gray
'--color-background': '28 28 30', // Dark background
'--color-text': '255 255 255' // White
})
};
// Theme component to apply the current theme
export function ThemeProvider({ children }: { children: React.ReactNode }) {
const { colorScheme } = useColorScheme();
return <>{children}</>;
}
Implementing Dark Mode
Setting Up Theme Provider
Create a theme context to manage the theme state:
// src/contexts/ThemeContext.tsx
import React, { createContext, useContext, useEffect, useState } from 'react';
import { useColorScheme as useDeviceColorScheme } from 'react-native';
import { useColorScheme, vars } from 'nativewind';
import { themes } from '../core/utilities/tailwind';
type ThemeContextType = {
theme: 'light' | 'dark';
setTheme: (theme: 'light' | 'dark' | 'system') => void;
isSystemTheme: boolean;
};
const ThemeContext = createContext<ThemeContextType | undefined>(undefined);
export function ThemeProvider({ children }: { children: React.ReactNode }) {
const deviceTheme = useDeviceColorScheme();
const [isSystemTheme, setIsSystemTheme] = useState(true);
const { setColorScheme } = useColorScheme();
const [theme, setThemeState] = useState<'light' | 'dark'>(
deviceTheme === 'dark' ? 'dark' : 'light'
);
const setTheme = (newTheme: 'light' | 'dark' | 'system') => {
if (newTheme === 'system') {
setIsSystemTheme(true);
const systemTheme = deviceTheme === 'dark' ? 'dark' : 'light';
setThemeState(systemTheme);
setColorScheme(systemTheme);
} else {
setIsSystemTheme(false);
setThemeState(newTheme);
setColorScheme(newTheme);
}
};
// Listen for device theme changes when using system theme
useEffect(() => {
if (isSystemTheme) {
const newTheme = deviceTheme === 'dark' ? 'dark' : 'light';
setThemeState(newTheme);
setColorScheme(newTheme);
}
}, [deviceTheme, isSystemTheme, setColorScheme]);
return (
<ThemeContext.Provider value={{ theme, setTheme, isSystemTheme }}>
<div style={themes[theme]}>{children}</div>
</ThemeContext.Provider>
);
}
export const useTheme = () => {
const context = useContext(ThemeContext);
if (context === undefined) {
throw new Error('useTheme must be used within a ThemeProvider');
}
return context;
};
Using the Theme Provider
Wrap your application with the ThemeProvider in your App.tsx
:
// App.tsx
import React from 'react';
import { SafeAreaView, StatusBar } from 'react-native';
import { ThemeProvider } from './src/contexts/ThemeContext';
import MainNavigation from './src/navigation/MainNavigation';
function App(): React.JSX.Element {
return (
<ThemeProvider>
<SafeAreaView className="flex-1 bg-background">
<StatusBar barStyle="dark-content" backgroundColor="transparent" translucent />
<MainNavigation />
</SafeAreaView>
</ThemeProvider>
);
}
export default App;
Theme Switching Component
Create a theme switcher component that allows users to toggle between light, dark, and system themes:
// src/components/ThemeSwitcher.tsx
import React from 'react';
import { View, Text, TouchableOpacity } from 'react-native';
import { useTheme } from '../contexts/ThemeContext';
import { cn } from '../core/utilities/tailwind';
export function ThemeSwitcher() {
const { theme, setTheme, isSystemTheme } = useTheme();
return (
<View className="p-4 rounded-lg bg-secondary">
<Text className="text-text font-bold mb-4">Theme Preferences</Text>
<View className="flex-row space-x-2">
<TouchableOpacity
className={cn(
'p-3 rounded-md flex-1 items-center',
!isSystemTheme && theme === 'light' ? 'bg-primary' : 'bg-secondary'
)}
onPress={() => setTheme('light')}
>
<Text
className={cn(
'font-medium',
!isSystemTheme && theme === 'light' ? 'text-white' : 'text-text'
)}
>
Light
</Text>
</TouchableOpacity>
<TouchableOpacity
className={cn(
'p-3 rounded-md flex-1 items-center',
!isSystemTheme && theme === 'dark' ? 'bg-primary' : 'bg-secondary'
)}
onPress={() => setTheme('dark')}
>
<Text
className={cn(
'font-medium',
!isSystemTheme && theme === 'dark' ? 'text-white' : 'text-text'
)}
>
Dark
</Text>
</TouchableOpacity>
<TouchableOpacity
className={cn(
'p-3 rounded-md flex-1 items-center',
isSystemTheme ? 'bg-primary' : 'bg-secondary'
)}
onPress={() => setTheme('system')}
>
<Text className={cn('font-medium', isSystemTheme ? 'text-white' : 'text-text')}>
System
</Text>
</TouchableOpacity>
</View>
</View>
);
}
Example Screen
Here’s an example of a screen that uses NativeWind classes and adapts to the theme:
// src/screens/HomeScreen.tsx
import React from 'react';
import { View, Text, ScrollView } from 'react-native';
import { ThemeSwitcher } from '../components/ThemeSwitcher';
import { cn } from '../core/utilities/tailwind';
export default function HomeScreen() {
return (
<ScrollView className="flex-1 bg-background p-4">
<Text className="text-text text-2xl font-bold mb-6">Welcome</Text>
<View className="bg-secondary p-4 rounded-lg mb-6">
<Text className="text-text">
This is a simple example of using NativeWind with dark mode support. Try switching between
light and dark themes!
</Text>
</View>
<View className="mb-6">
<Text className="text-text font-semibold mb-2">Primary Button</Text>
<View className={cn('bg-primary p-4 rounded-lg items-center')}>
<Text className="text-white font-medium">Click Me</Text>
</View>
</View>
<View className="mb-6">
<Text className="text-text font-semibold mb-2">Secondary Button</Text>
<View className={cn('bg-secondary border border-text/10 p-4 rounded-lg items-center')}>
<Text className="text-text font-medium">Cancel</Text>
</View>
</View>
<ThemeSwitcher />
</ScrollView>
);
}
Using Tailwind Merge for Dynamic Classnames
The cn
utility function we defined earlier helps combine and manage conditional classes. Here’s a practical example:
// src/components/Button.tsx
import React from 'react';
import { TouchableOpacity, Text, TouchableOpacityProps } from 'react-native';
import { cn } from '../core/utilities/tailwind';
interface ButtonProps extends TouchableOpacityProps {
variant?: 'primary' | 'secondary' | 'outline';
size?: 'sm' | 'md' | 'lg';
label: string;
}
export default function Button({
variant = 'primary',
size = 'md',
label,
className,
...props
}: ButtonProps) {
return (
<TouchableOpacity
className={cn(
'rounded-md items-center justify-center',
// Variant styles
variant === 'primary' && 'bg-primary',
variant === 'secondary' && 'bg-secondary',
variant === 'outline' && 'bg-transparent border border-primary',
// Size styles
size === 'sm' && 'px-3 py-1.5',
size === 'md' && 'px-4 py-2.5',
size === 'lg' && 'px-6 py-3',
// Allow passing additional classes
className
)}
{...props}
>
<Text
className={cn(
'font-medium',
variant === 'primary' && 'text-white',
variant === 'secondary' && 'text-text',
variant === 'outline' && 'text-primary'
)}
>
{label}
</Text>
</TouchableOpacity>
);
}
Platform-Specific Styling
NativeWind supports platform-specific styling through custom utility functions:
// tailwind.config.js
const { platformSelect } = require('nativewind/theme');
module.exports = {
// ... your existing config
theme: {
extend: {
colors: {
// ... your existing colors
accent: platformSelect({
ios: '#007AFF', // iOS blue
android: '#6200EE', // Material purple
default: '#0077CC' // Default for other platforms
})
}
}
}
};
You can then use it in your components:
<View className="bg-accent p-4 rounded-lg">
<Text className="text-white">I have platform-specific colors</Text>
</View>
Conclusion
You’ve now set up NativeWind with dark mode support in your React Native application. You can easily:
- Use Tailwind CSS utility classes for styling
- Switch between light and dark themes
- Use conditional class application with the
cn
utility - Combine classes efficiently with tailwind-merge
- Create reusable components with theme awareness
Remember to run npx tailwindcss init
to initialize your Tailwind configuration if you haven’t already. This setup provides a solid foundation for building a theme-aware React Native application with the power of Tailwind CSS.
Subscribe now!
Get latest news in regards to tech.
We wont spam you. Promise.
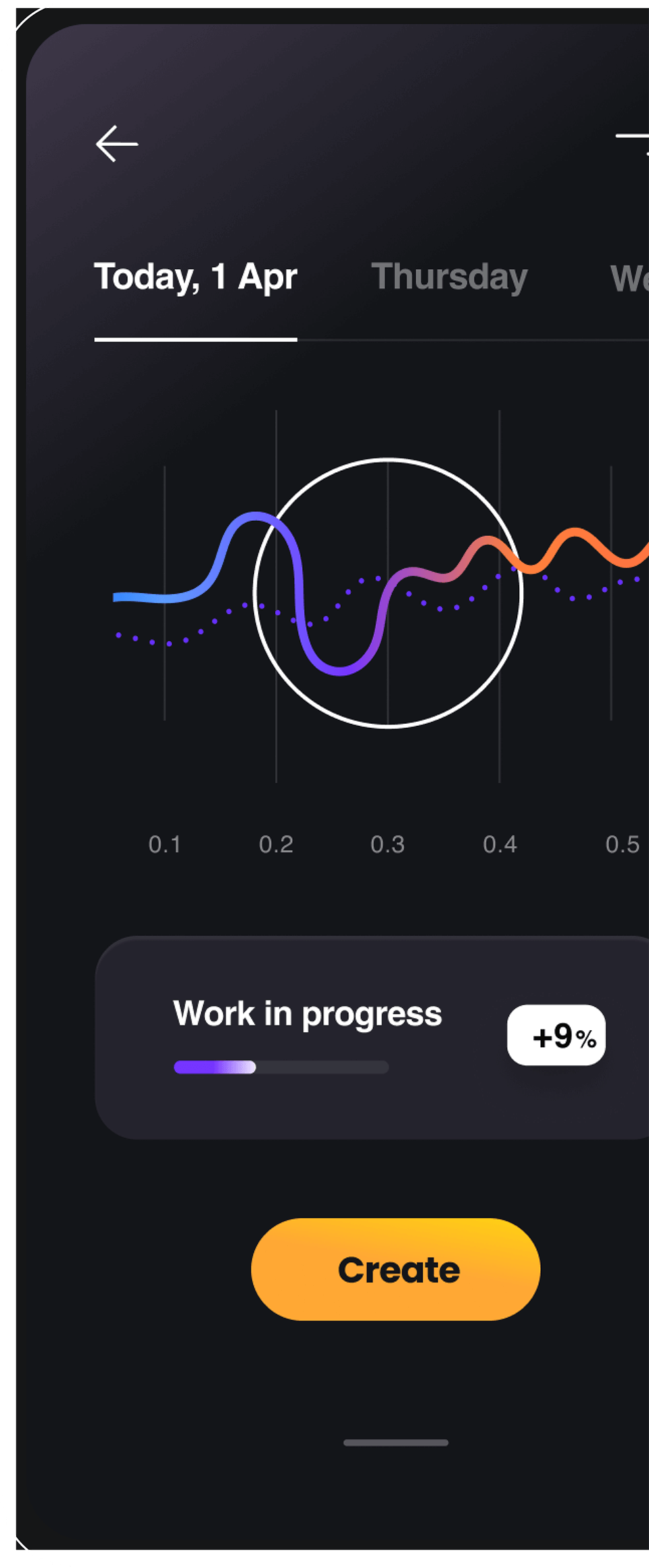
© 2025 Otherside Limited. All rights reserved.